Flags with Rectangles: Difference between revisions
Jump to navigation
Jump to search
No edit summary Tag: Reverted |
No edit summary Tag: Manual revert |
||
(3 intermediate revisions by the same user not shown) | |||
Line 1: | Line 1: | ||
<pre id='shellbody' data-qtp='canvas'></pre> | <pre id='shellbody' data-qtp='canvas'></pre> | ||
You can dive right in and start programming - or you can watch this worked example: [[Worked example Benin|Flag of Benin]] | |||
*You will need to use the method [https://developer.mozilla.org/en-US/docs/Web/API/CanvasRenderingContext2D/fillRect fillRect] | |||
*You will need to use the property [https://developer.mozilla.org/en-US/docs/Web/API/CanvasRenderingContext2D/fillStyle fillStyle] | |||
<div class='qu' data-width=" | ==Libya== | ||
<div class='qu' data-width="150" data-height="100"> | |||
The flag of Libya, until 2011, was a simple green rectangle. | |||
Try the program as it is given, then change it so that the green rectangle is 150 wide. | |||
[[Image:flaglibya.png|border]] | |||
<pre class='usr'> | <pre class='usr'> | ||
function drawFlag(ctx) | function drawFlag(ctx) | ||
{ | { | ||
ctx.fillStyle = ' | ctx.fillStyle = 'green'; | ||
ctx.fillRect(0,0, | ctx.fillRect(0,0,75,100); | ||
} | } | ||
</pre> | </pre> | ||
Line 23: | Line 23: | ||
ctx.fillRect(0,0,150,100); | ctx.fillRect(0,0,150,100); | ||
} | } | ||
</pre> | |||
</div> | |||
==France== | |||
<div class=qu data-width="150" data-height="100"> | |||
The flag of France is blue, white and red. | |||
The code given draws only one of the three rectangles required and it is in the wrong place.<br/> | |||
[[Image:flag_guide_france.png|border]] | |||
<pre class=usr> | |||
function drawFlag(ctx) | |||
{ | |||
ctx.fillStyle = 'blue'; | |||
ctx.fillRect(50,0,50,100); | |||
} | |||
</pre> | |||
<pre class=ans>function drawFlag(ctx) | |||
{ | |||
ctx.fillStyle = 'blue'; | |||
ctx.fillRect(0,0,50,100); | |||
ctx.fillStyle = 'white'; | |||
ctx.fillRect(50,0,50,100); | |||
ctx.fillStyle = 'red'; | |||
ctx.fillRect(100,0,50,100); | |||
} | |||
</pre> | |||
</div> | |||
==Germany== | |||
<div class=qu data-width=150 data-height=90> | |||
The flag of Germany is black, red and yellow. | |||
Only one of the three rectangles has been drawn - and that one is in the wrong place.<br/> | |||
[[Image:flaggermany.png|border]] <pre class=usr> | |||
function drawFlag(ctx) | |||
{ | |||
ctx.fillStyle = 'yellow'; | |||
ctx.fillRect(0,0,150,30); | |||
} | |||
</pre> | |||
<pre class=ans> | |||
function drawFlag(ctx) | |||
{ | |||
ctx.fillStyle = 'black'; | |||
ctx.fillRect(0,0,150,30); | |||
ctx.fillStyle = 'red'; | |||
ctx.fillRect(0,30,150,30); | |||
ctx.fillStyle = 'yellow'; | |||
ctx.fillRect(0,60,150,30); | |||
} | |||
</pre> | |||
</div> | |||
==Switzerland== | |||
<div class=qu width='100' height='100' | |||
className="Raster" level='1'> | |||
The flag of Switzerland is red with a white cross in the center. | |||
The background has been filled in. Use white rectangles to draw the cross.<br/> | |||
[[Image:swissflag.png|border]] <pre class=usr>function drawFlag(ctx) | |||
{ | |||
ctx.fillStyle = 'red'; | |||
ctx.fillRect(0,0,100,100); | |||
} | |||
</pre> | |||
<pre class=ans> | |||
function drawFlag(ctx) | |||
{ | |||
ctx.fillStyle = 'red'; | |||
ctx.fillRect(0,0,100,100); | |||
ctx.fillStyle = 'white'; | |||
ctx.fillRect(10,40,80,20); | |||
ctx.fillRect(40,10,20,80); | |||
} | |||
</pre></div> | |||
==United Arab Emirates== | |||
<div class=qu data-width='200' data-height='99'> | |||
*The flag of United Arab Emirates has a red bar taking one quarter of the rectangle. | |||
*The rectangle is 200 by 99. | |||
[[Image:Flaguaeplain.png|border]] | |||
<div class='dhint' title="How to draw a circle"> | |||
</div> | |||
<pre class=usr> | |||
function drawFlag(ctx) | |||
{ | |||
ctx.fillStyle = 'white'; | |||
ctx.fillRect(50,0,150,33); | |||
ctx.fillStyle = 'red'; | |||
} | |||
</pre> | |||
<pre class=ans> | |||
function drawFlag(ctx) | |||
{ | |||
ctx.fillStyle = 'red'; | |||
ctx.fillRect(0,0,50,99); | |||
ctx.fillStyle = 'black'; | |||
ctx.fillRect(50,0,150,33); | |||
ctx.fillStyle = 'white'; | |||
ctx.fillRect(50,33,150,33); | |||
ctx.fillStyle = 'green'; | |||
ctx.fillRect(50,66,150,33); | |||
} | |||
</pre> | </pre> | ||
</div> | </div> |
Latest revision as of 21:33, 28 August 2023
You can dive right in and start programming - or you can watch this worked example: Flag of Benin
1) Libya
The flag of Libya, until 2011, was a simple green rectangle.
Try the program as it is given, then change it so that the green rectangle is 150 wide.
Input
xxxxxxxxxx
function drawFlag(ctx)
{
ctx.fillStyle = 'green';
ctx.fillRect(0,0,75,100);
}
Output
function drawFlag(ctx) { ctx.fillStyle = 'green'; ctx.fillRect(0,0,75,100); }
function drawFlag(ctx) { ctx.fillStyle = 'green'; ctx.fillRect(0,0,150,100); }
2) France
The flag of France is blue, white and red.
The code given draws only one of the three rectangles required and it is in the wrong place.
Input
xxxxxxxxxx
function drawFlag(ctx)
{
ctx.fillStyle = 'blue';
ctx.fillRect(50,0,50,100);
}
Output
function drawFlag(ctx) { ctx.fillStyle = 'blue'; ctx.fillRect(50,0,50,100); }
function drawFlag(ctx) { ctx.fillStyle = 'blue'; ctx.fillRect(0,0,50,100); ctx.fillStyle = 'white'; ctx.fillRect(50,0,50,100); ctx.fillStyle = 'red'; ctx.fillRect(100,0,50,100); }
3) Germany
The flag of Germany is black, red and yellow.
Only one of the three rectangles has been drawn - and that one is in the wrong place.
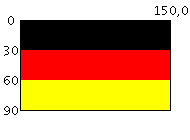
Input
xxxxxxxxxx
function drawFlag(ctx)
{
ctx.fillStyle = 'yellow';
ctx.fillRect(0,0,150,30);
}
Output
function drawFlag(ctx) { ctx.fillStyle = 'yellow'; ctx.fillRect(0,0,150,30); }
function drawFlag(ctx) { ctx.fillStyle = 'black'; ctx.fillRect(0,0,150,30); ctx.fillStyle = 'red'; ctx.fillRect(0,30,150,30); ctx.fillStyle = 'yellow'; ctx.fillRect(0,60,150,30); }
4) Switzerland
The flag of Switzerland is red with a white cross in the center.
The background has been filled in. Use white rectangles to draw the cross.
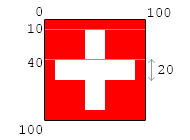
Input
xxxxxxxxxx
function drawFlag(ctx)
{
ctx.fillStyle = 'red';
ctx.fillRect(0,0,100,100);
}
Output
function drawFlag(ctx) { ctx.fillStyle = 'red'; ctx.fillRect(0,0,100,100); }
function drawFlag(ctx) { ctx.fillStyle = 'red'; ctx.fillRect(0,0,100,100); ctx.fillStyle = 'white'; ctx.fillRect(10,40,80,20); ctx.fillRect(40,10,20,80); }
5) United Arab Emirates
- The flag of United Arab Emirates has a red bar taking one quarter of the rectangle.
- The rectangle is 200 by 99.
Input
xxxxxxxxxx
function drawFlag(ctx)
{
ctx.fillStyle = 'white';
ctx.fillRect(50,0,150,33);
ctx.fillStyle = 'red';
}
Output
function drawFlag(ctx) { ctx.fillStyle = 'white'; ctx.fillRect(50,0,150,33); ctx.fillStyle = 'red'; }
function drawFlag(ctx) { ctx.fillStyle = 'red'; ctx.fillRect(0,0,50,99); ctx.fillStyle = 'black'; ctx.fillRect(50,0,150,33); ctx.fillStyle = 'white'; ctx.fillRect(50,33,150,33); ctx.fillStyle = 'green'; ctx.fillRect(50,66,150,33); }